getting started
premium components
deprecated components
Creating a report
The easiest way to create a report is using our Cloud Editor, that lets you write JSON and see your PDF side-by-side. You'll get code-completion for all the fields, and detailed error messages rendered in a visual format. Once you've perfected your report, you can then call the API programmatically to integrate it into your workflow or application.
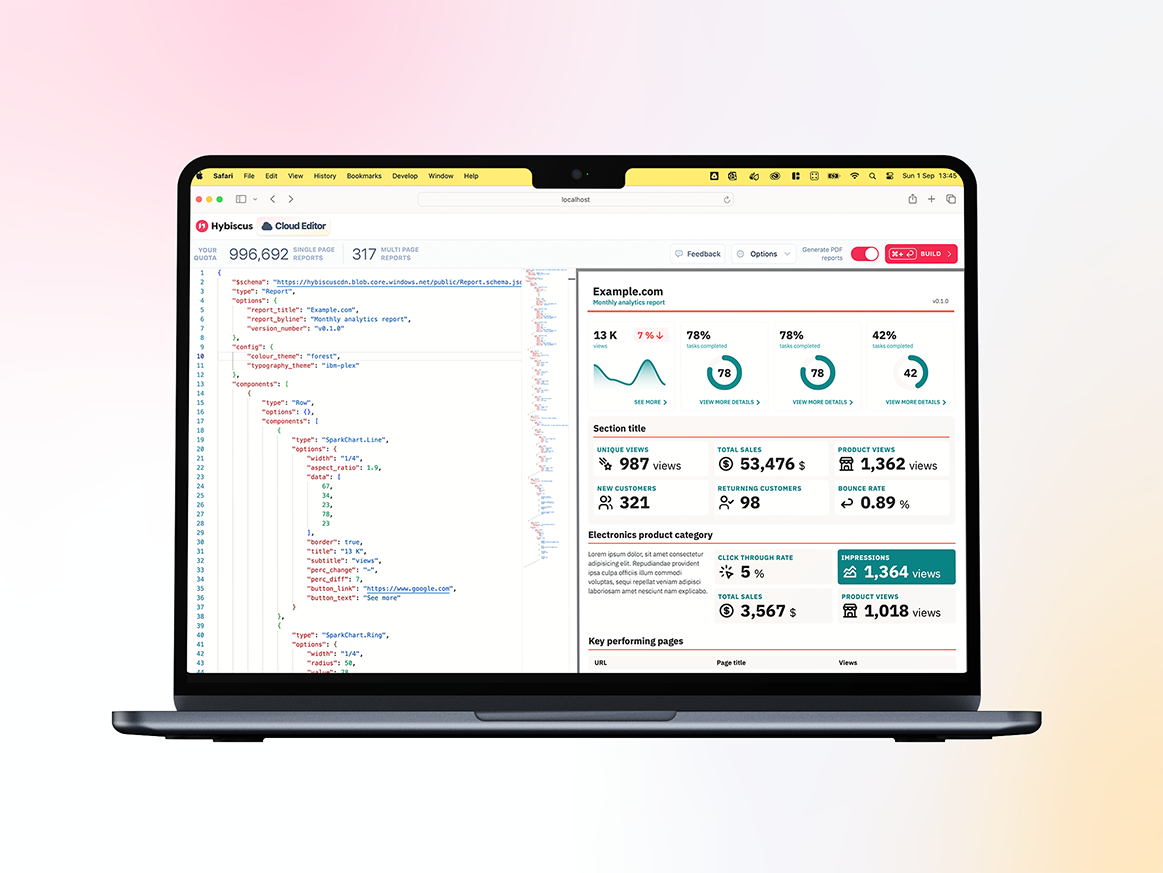
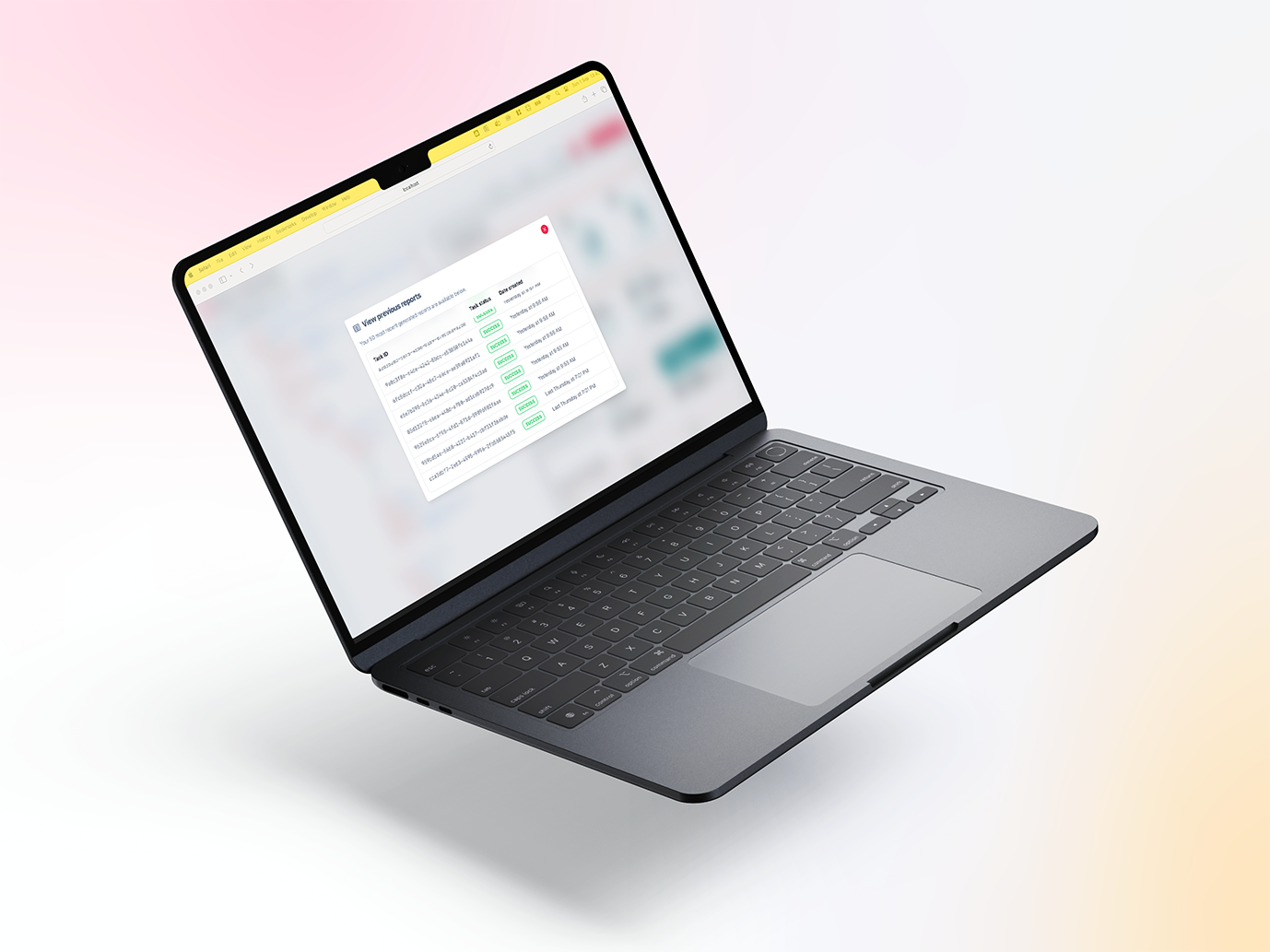
To get started with using the API directly, you'll need to make HTTP requests to the REST API. You can do this using your programming language of choice, or using tools such as Postman, Insomnia, or Bruno. We also provide a developer SDK for NodeJS, which you can learn more about here.
Steps to make a report
The process for generating a PDF report is 3 steps:
Submit report job
Make a HTTP POST request, with a JSON body specifying the content of your PDF. A task ID will be returned to you if successful.
Check job status
Query the task status endpoint using your task ID to check when your report is ready.
Download report
Once ready, download the PDF using the get report endpoint.
Authentication
To use any of the API endpoints, you must authenticate yourself using the API key provided in the dashboard. You can generate your API key by going to the Dashboard from the upper right hand corner, as well as viewing your existing API keys there.
The API key may either be placed in the header of your HTTP request under
the name X-API-KEY
, or you may pass it as a parameter in the query string
using the key api_key
. For more details view the authentication page.
Example
In this example, we will go through the steps using Python to generate a PDF report.
Submit report job
First, we will make a HTTP POST request to https://api.hybiscus.dev/api/v1/build-report
endpoint. The body of this request is a JSON definition used to define the report.
The structure is fairly simple, you start with an object, in which you have at
least 3 keys:
type
options
components
The type
is set to Report
as we're creating a PDF report (more options will
come in future), while the options
keys provides specific options for this
specific component. Lastly, the components
key is an array of objects with the same
schema as we've just described. You just need to change the type
to use different components,
to add content to your report.
Components are the building blocks of a report. Some components help with layout such as the Section, where as others allow you to add content such as the Card component. You can nest components as deep as you like, or add them side by side together in the array.
import requests
import json
URL = "https://api.hybiscus.dev/api/v1/build-report"
REPORT_SCHEMA = {
"type": "Report",
"options": {
"report_title": "The report",
"report_byline": "Yet another byline",
"version_number": "0.1b"
},
"components": [
{
"type": "Section",
"options": {
"section_title": "Section one",
},
"components": [
{
"type": "Card",
"options": {
"title": "Loremp ipsum",
"value": 700,
"units": "rpm"
}
}
]
}
]
}
response = requests.post(
url=URL,
headers={
"X-API-KEY": "<<YOUR_API_KEY>>"
},
data=json.dumps(REPORT_SCHEMA)
)
print(response.content)
>>> {"task_id":"ca173e1d-f487-4db2-891b-e347d92df6e2","status":"QUEUED"}
If your request was successful, the server will respond with the task_id
and the status
.
Check job status
To get the latest status of your task, make a GET request to the API endpoint
https://api.hybiscus.dev/api/v1/get-task-status
, specifying the
task_id
as a parameter in the query string.
import requests
URL = "https://api.hybiscus.dev/api/v1/get-task-status"
response = requests.get(
url=URL,
headers={
"X-API-KEY": "<<YOUR_API_KEY>>"
},
params={"task_id": "<<YOUR_TASK_ID_HERE>>"}
)
print(response.content)
>>> {"status":"SUCCESS"}
Download report
If the task status is SUCCESS
, you can now download your report from the API
endpoint https://api.hybiscus.dev/api/v1/get-report
. As this URL will
simply return the PDF report, you can even use this URL in your web browser.
Since web browsers do not let you specify headers when navigating to a URL, we can instead make use of the query parameters to authenticate ourselves.
Thus, the following URL structure can be used to download our PDF:
https://api.hybiscus.dev/api/v1/get-report?task_id=<<TASK_ID>>&api_key=<<API_KEY>>
JSON schema
When developing your report, you may find it useful to refer to the JSON schema which is publically available here.
Missing something?
Hybiscus is continuously improving and adding new features. If you think we are missing a critical feature, please do not hesitate to contact us and offer your feedback at support@hybiscus.dev