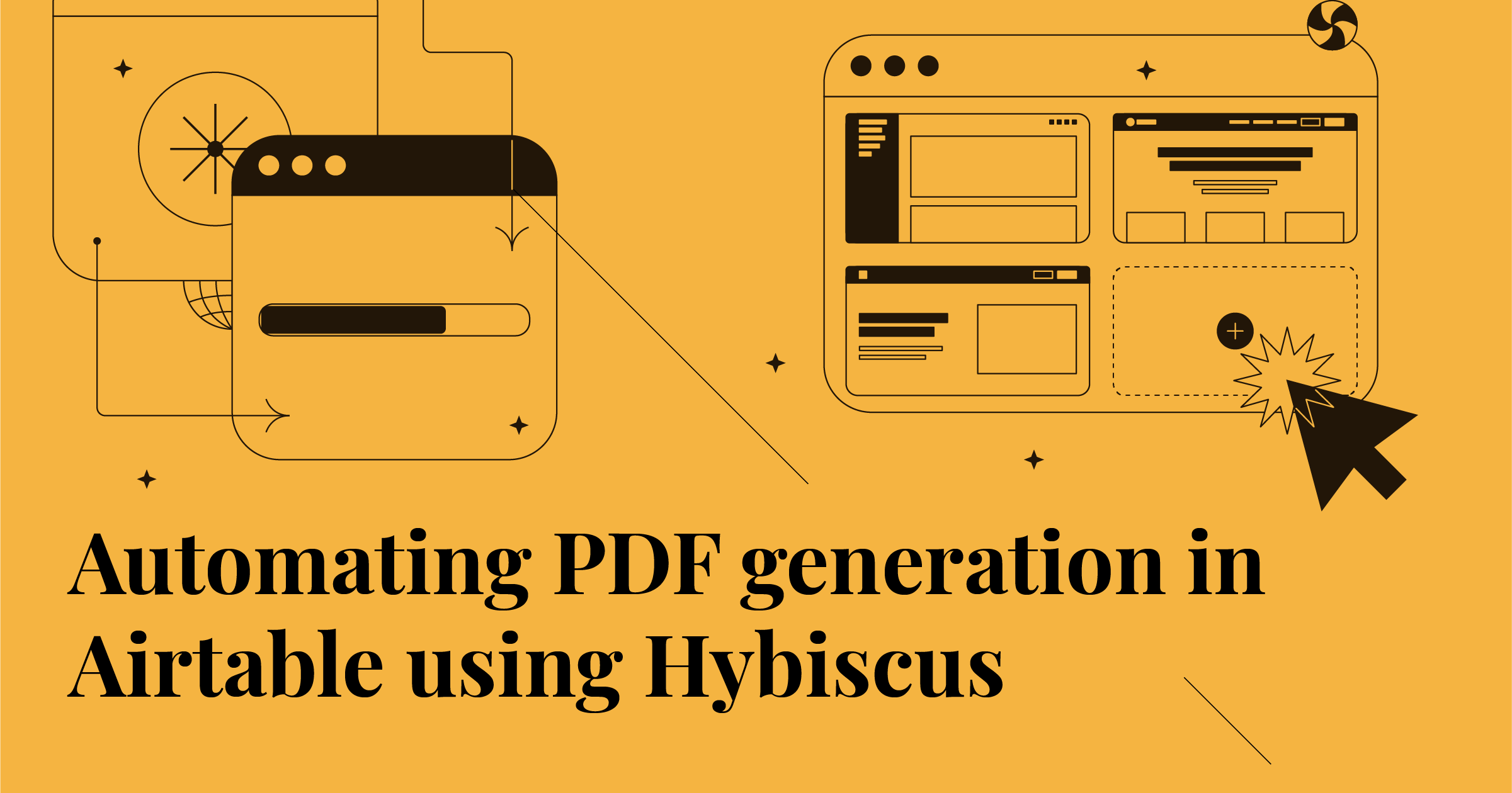
Automating PDF generation from Airtable using Hybiscus
Use Airtable scripts to call the Hybiscus API to generate PDF reports automatically
One of the great things about the Hybiscus API is that it can be integrated with any other service that can make an API call. This makes Hybiscus compatible with a wide range of automation solutions out there, as well as some great no code tools such as Airtable.
Today, we'll take a look at an example of how we can very easily set this up inside Airtable. Below is an example table, from which we would like to generate a PDF report.
To begin, you'll first need an Hybiscus account, and your API key which you can get from here.
Setup an Airtable script
To enable scripting in Airtable, you'll need to add an extension from the Extensions link tab on the top right side. From there, search for the Scripting extension.
The scripting extension lets you write JavaScript to automate the process of extracting data from your table, and formatting to make an API call, in this case to Hybiscus.
The first bit of code you'll need to write will be to extract the data
from your table, and assemble a list of headings and rows for the Hybiscus
table. You'll need to replace the value of TABLE_NAME
with your actual
table name.
const TABLE_NAME = "Competitors";
// Extract the table data from Airtable
let table = base.getTable(TABLE_NAME);
let query = await table.selectRecordsAsync({fields: table.fields});
// Get the list of headings / columns from the table
let headings = table.fields.map(f => f.name);
let rows = [];
let row = [];
// Loop over the rows / records of the table
for (const record of query.records) {
row = [];
// Loop over the columns / fields of the table
for (const field of table.fields) {
let value = record.getCellValue(field.id);
// Over here, we're checking if the column is the website column
// and selectively formatting it as a hyperlink
if (field.name === "Website") {
row.push(`<a href="${value}">${value}</a>`);
} else {
row.push(value);
}
}
// Add the row to the list of rows
rows.push(row);
}
With the rows and headings constructed, we can now begin to setup the JSON report schema for Hybiscus:
// Assemble Hybiscus PDF report schema
const reportSchema = {
"type": "Report",
"options": {
"report_title": "Market analysis",
"report_byline": "Monthly market reports",
"version_number": ""
},
"config": {
"colour_theme": "candy",
"typography_theme": "jost"
},
"components": [
{
"type": "Section",
"options": {
"section_title": "Competitors",
},
"components": [
{
"type": "Table",
"options": {
"headings": headings,
"rows": rows
}
}
]
}
]
}
Now that the report schema is ready, we can make the API call to Hybiscus. This part will make the call to Hybiscus, and then return a link in Airtable which you can click to open the PDF.
// Assemble API request
const API_KEY = '<<<REPLACE_WITH_YOUR_API_KEY>>>';
let response = await remoteFetchAsync('https://api.hybiscus.dev/api/v1/build-report', {
method: 'POST',
body: JSON.stringify(reportSchema),
headers: {
'Content-Type': 'application/json',
'X-API-KEY': API_KEY
}
});
let jsonResponse = await response.json();
console.log(jsonResponse);
let taskID = jsonResponse.task_id;
let reportURL = `https://api.hybiscus.dev/api/v1/get-report?task_id=${taskID}&api_key=${API_KEY}`;
output.markdown(`[Report URL](${reportURL})`);
Note This script will return the link to the PDF report immediately, even though the report may not yet be ready. This is because Airtable scripting does not support a method to run a function on an interval, and check when the report is ready. Hybiscus PDF reports typically take between 2-4 s to generate.
All the PDF reports you generate with your account will also be accessible from the Hybiscus dashboard.
You can find the complete script over on our GitHub repo, and the link to download the PDF here.